Types are nice, infinite recursion edition
Playing around on hackerrank to prepare for an interview, I had some fun solving tree problems using recursion.
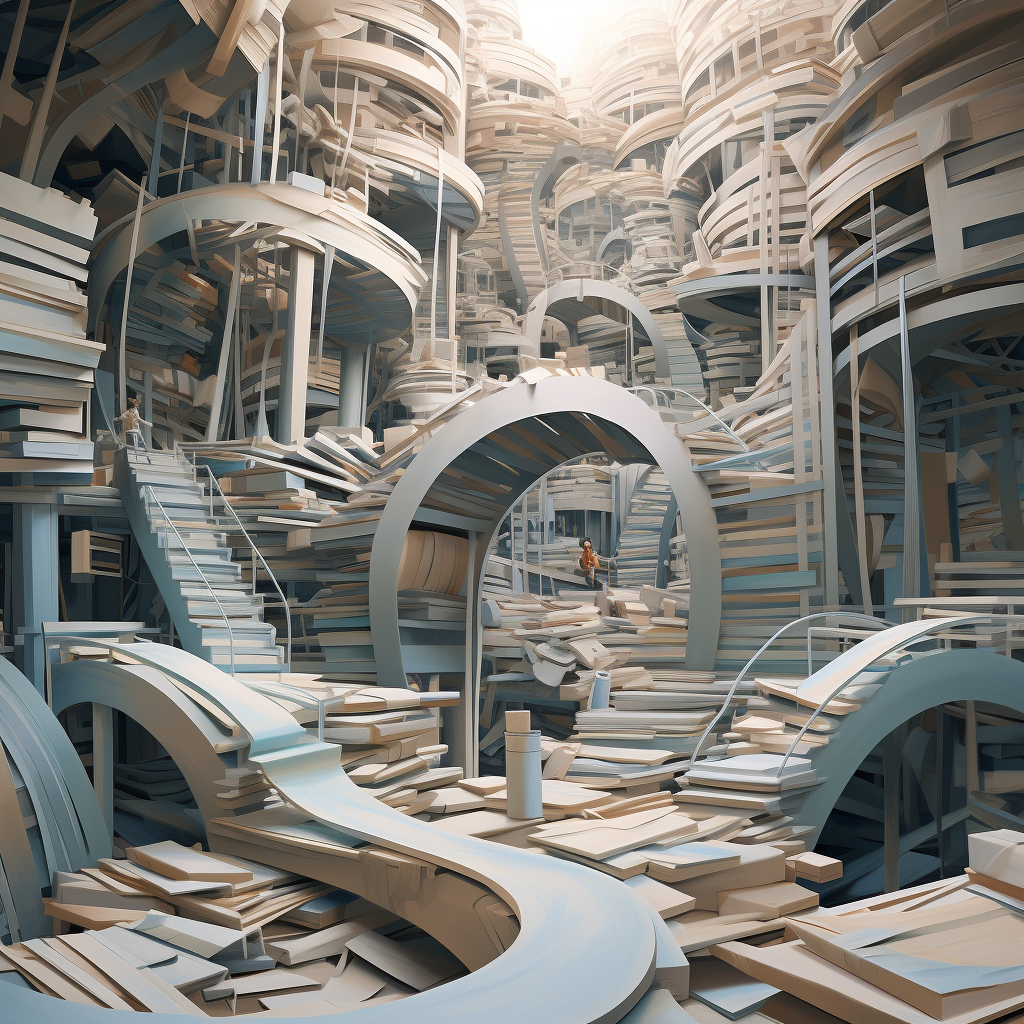
RangeError: Maximum call stack size exceeded
Can you spot what's wrong here?
/*
Node is defined as
var Node = function(data) {
this.data = data;
this.left = null;
this.right = null;
}
*/
function treeHeight(root) {
if (!root) { return 0 }
return 1 + Math.max(treeHeight(root.left), treeHeight(root).right)
}
Absolutely, yes, line 12:
return 1 + Math.max(treeHeight(root.left), treeHeight(root).right)
A misplaced parenthesis, meaning this recursive function has no exit condition. Instead of calling itself with both root.left
and root.right
, it will call itself with root.left
and root
, the input parameter. Until it blows the stack.
And since a number
has no right
property, proper typing would have easily avoided that.
¯\_(ツ)_/¯
function treeHeight(root) {
if (!root) { return 0 }
return 1 + Math.max(treeHeight(root.left), treeHeight(root.right))
}
Comments ()