What do to when your Node.js code crashes because of a circular JSON
You're trying to figure out what some object looks like, and since you're not running your code locally you don't have access to a proper debugger. So you print it. And your whole Node.js app crashes. What do you do?
It's pretty certainly happened to every one of us at some point or another. Debugging our Node.js code, trying to surface some errors through our logs or observability tools, only to be hit by this:
TypeError: Converting circular structure to JSON
at JSON.stringify (<anonymous>)
at...
This means that the JSON we're trying to print contains references to itself or other parts of itself. Circular references. Like this:
const nestedObject = { prop1: "value" }
let circularObject: any = {};
circularObject.prop1 = nestedObject;
circularObject.prop1.subprop1 = nestedObject;
Now, while some will suggest some custom replacers for dear JSON.stringify
, looping through our objects to eliminate the culprit duplicate reference, I am a lazy one. A lazy one who stricly adheres by the following rule: The best code is the code we don't write (see Jeff Atwood's and Rich Skrenta's takes on the matter).
And it just so happens that Node.js' util
module has something that already does the job well enough: inspect
.
Here's how to use it:
import { inspect } from 'util'
const nestedObject = { prop1: "value" }
let circularObject: any = {};
circularObject.prop1 = nestedObject;
circularObject.prop1.subprop1 = nestedObject;
console.log(inspect(circularObject))
Yes, that is it! Now you can happily debug your code, circular objects be damned.
Take aways
Three simple rules to remember:
- The best code is the code you don't write.
- Don't reinvent the wheel (unless you plan on learning more about wheels).
- And focus on what actually brings value to your product! Or your customer. Or what brings you joy. Whatever rocks your boat.
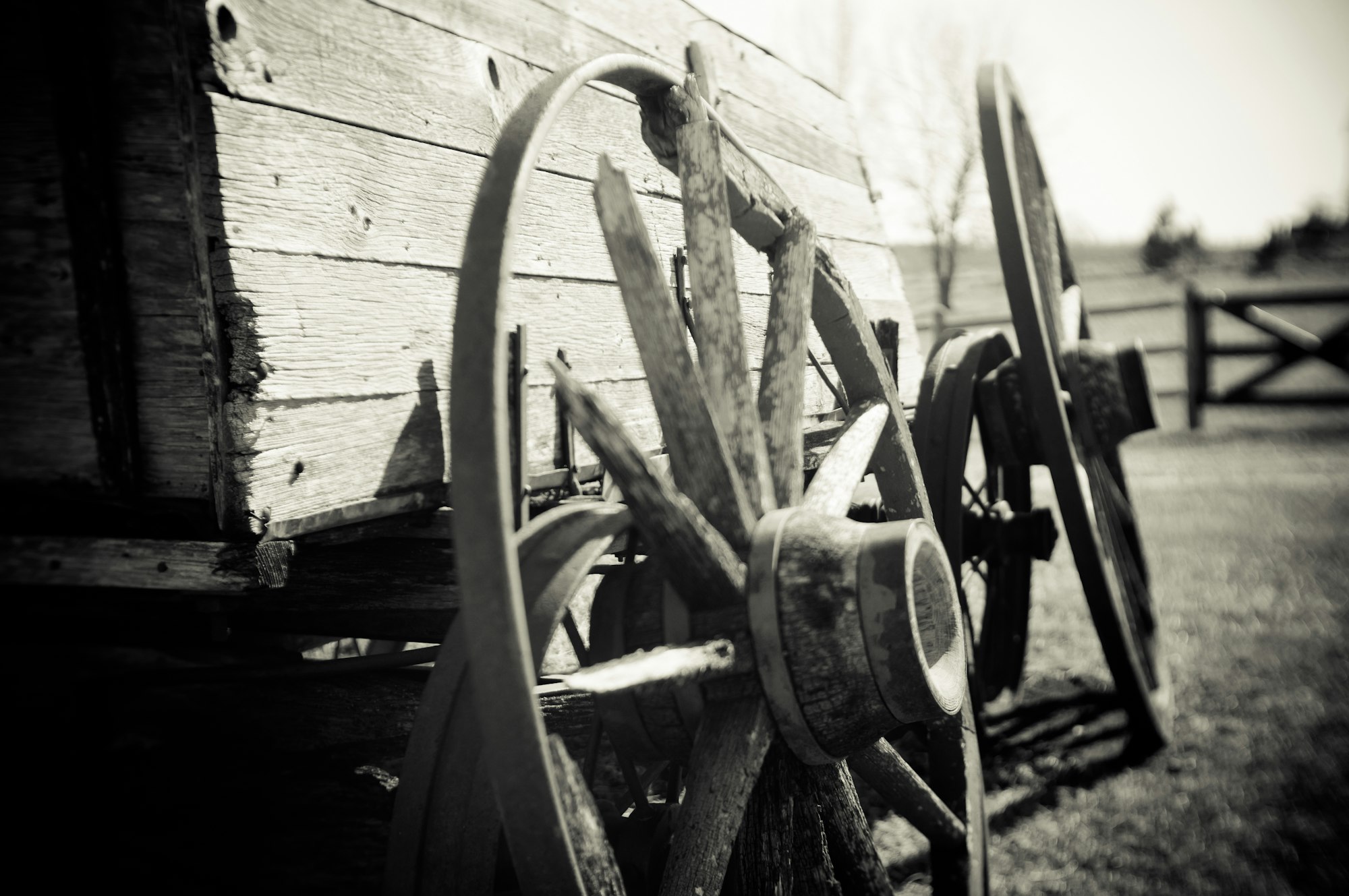
Comments ()